Combobox
Combobox is an input plus a listbox that appears beneath it. It searches and filters down options, allowing the user to select.
- To filter down a large list of options
- To make a selection from a list of options
- To pick an option from a list on mobile devices, use Select instead
How to use it
Default
By default, the Combobox presents a list of suggested options upon value entry.
Autocomplete
In conjunction with user-provided filtering, the isAutocomplete
prop
highlights an option for selection.
Disabled
A disabled Combobox prevents user interaction. It doesn’t appear in the tab order, can’t receive focus, and may not be read aloud by a screenreader.
Grouped options
Use grouped options for categorization.
Hidden label
Combobox labels can be hidden.
Hint text
Hint text gives further clarification.
Media
Media elements add even more context to a Combobox and its options.
Multiselect
Use the isMultiselectable
prop to allow multiple selections.
Multiselect tags
Follow Avatar guidelines by using
Option tagProps
to alter the appearance of multiselect tags.
Nested
A Combobox listbox can contain nested levels for additional categorization of options.
Search
Combobox can be used to provide search suggestions.
Select only
Add isEditable={false}
to make Combobox behave like a native <select>
.
Size
Combobox can be default or compact in size.
Validation
Show success, warning, and error validation messages with the Message component.
How to use it well
Narrow down options
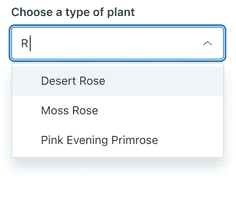
Combobox is useful for lists that are too long to read in full (for example, company employees) or that accumulate items over time (for example, an inventory).
Apply icons consistently
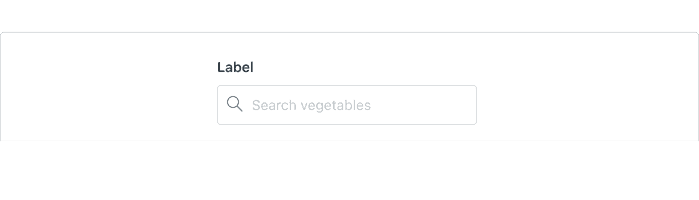
Always place the magnifying glass icon at the start of the input when using the Combobox for search.
Configuration
- Name8.76.4•View source•View on npm
- Installnpm install @zendeskgarden/react-dropdowns.next
- Depsnpm install react react-dom styled-components @zendeskgarden/react-theming
- Importimport { Combobox, Field, Hint, Label, Message, OptGroup, Option, Tag } from '@zendeskgarden/react-dropdowns.next'
API
The Combobox component follows this structure:
<Field>
<Label />
<Combobox>
<Option />
<Option />
<OptGroup>
<Option />
</OptGroup>
{/* etc. */}
</Combobox>
</Field>
Use Array.map
to iterate over options.
const OPTIONS = [
{ value: 'Sunflower' },
{
label: 'More flowers',
options: [
{ value: 'Violet' },
{ value: 'Daisy' },
]
}
]
<Combobox>
{OPTIONS.map(option =>
option.options ? (
<OptGroup key={option.label} label={option.label}>
{option.options.map(groupOption => (
<Option key={groupOption.value} {...groupOption} />
))}
</OptGroup>
) : (
<Option key={option.value} {...option} />
)
)}
</Combobox>
Match Option
and OptGroup
prop signatures when using wrapper components.
const OptionComponent = forwardRef((props, ref) => (
<Option {...props} ref={ref} />
))
const OptGroupComponent = forwardRef((props, ref) => (
<OptGroup {...props} ref={ref} />
))
<Combobox>
<OptGroupComponent label="Flowers">
<OptionComponent value="Violet" />
<OptionComponent value="Sunflower" />
<OptionComponent value="Daisy" />
</OptGroupComponent>
</Combobox>
Combobox
Extends HTMLAttributes<HTMLDivElement>
Nest a Combobox within a Field component.
Prop name | Type | Default | Description |
---|---|---|---|
activeIndex | number | – | Sets the currently active option index in a controlled combobox |
defaultActiveIndex | number | – | Sets the default active option index in an uncontrolled combobox |
defaultExpanded | boolean | – | Determines default listbox expansion in an uncontrolled combobox |
endIcon | ReactElement<any, string | JSXElementConstructor<any>> | – | Accepts an "end" icon to display |
focusInset | boolean | – | Applies inset box-shadow styling on focus |
id | string | – | Identifies the combobox |
inputProps | InputHTMLAttributes<HTMLInputElement> | – | Passes HTML attributes to the input element |
inputValue | string | – | Sets the input value in a controlled combobox |
isAutocomplete | boolean | – | Indicates that the combobox provides autocompletion |
isBare | boolean | – | Removes borders and padding |
isCompact | boolean | – | Applies compact styling |
isDisabled | boolean | – | Indicates that the combobox is not interactive |
isEditable | boolean | true | Determines whether the combobox is editable or select-only |
isExpanded | boolean | – | Determines listbox expansion in a controlled combobox |
isMultiselectable | boolean | – | Determines whether multiple options can be selected |
listboxAppendToNode | Element | DocumentFragment | – | Appends the lisbox to the element provided |
listboxAriaLabel | string | – | Specifies the listbox aria-label |
listboxMaxHeight | string | '400px' | Sets the max-height of the listbox |
listboxMinHeight | string | null | – | Overrides the min-height of the listbox |
listboxZIndex | number | 1000 | Sets the z-index of the listbox |
maxHeight | string | – | Overrides the max-height of the combobox |
maxTags | number | 4 | Determines the maximum number of tags displayed when a multiselectable combobox is collapsed |
onChange |